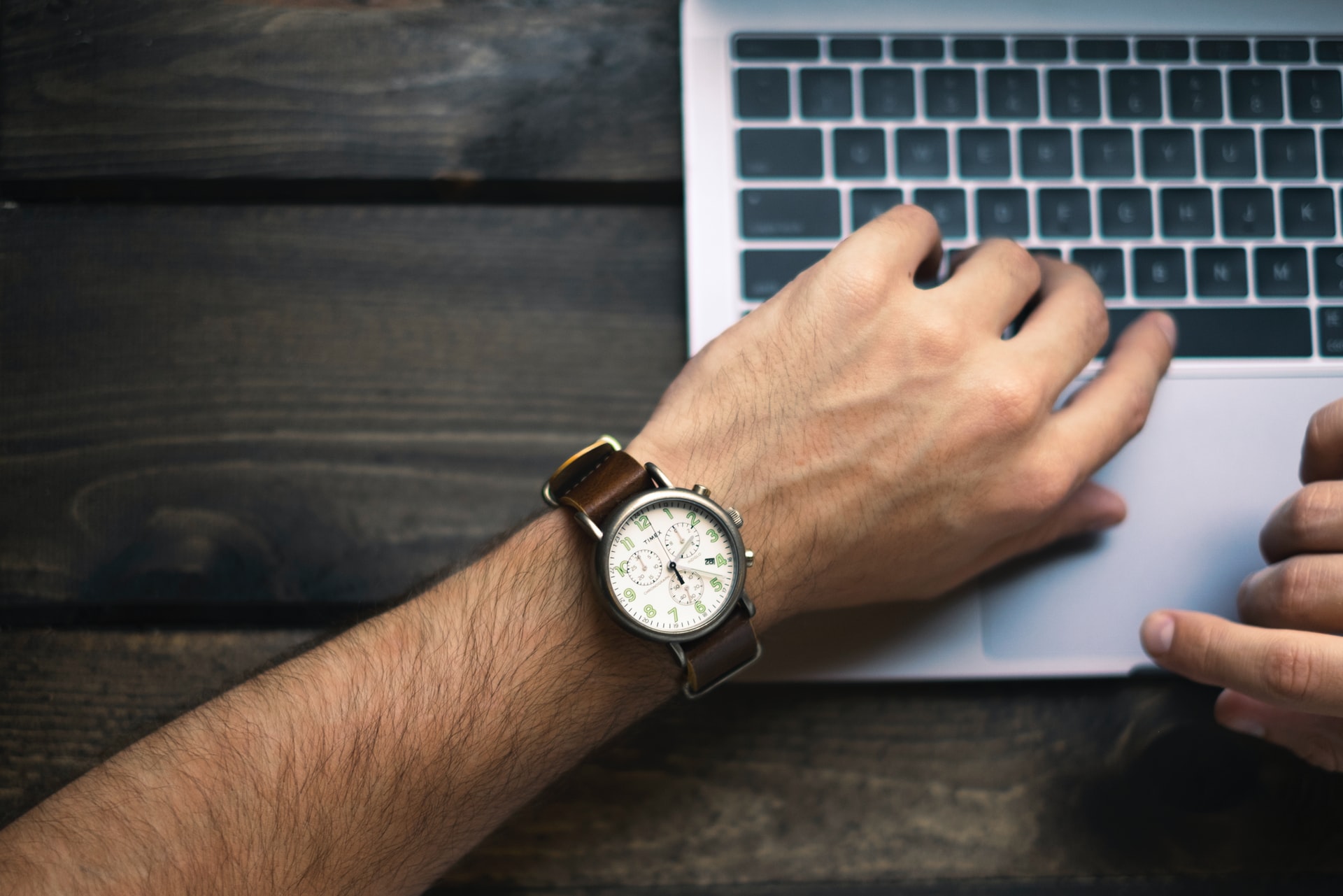
WordPress stores only the registration date for users. So what if your system needs to know the last login date for a user. Well there is no in-built functionality available to get this. But we can use login hook and a metadata to accomplish this.
First we need to add a function for this login hook. Lets say the function is “set_last_login”. This will be used to store the login time when a user will log in.
<?php //associating a function to login hook add_action('wp_login', 'set_last_login'); //function for setting the last login function set_last_login($login) { $user = get_userdatabylogin($login); //add or update the last login value for logged in user update_usermeta( $user->ID, 'last_login', current_time('mysql') ); } ?>As we have stored the last login time, now we can have a function to get the same.
<?php //function for getting the last login function get_last_login($user_id) { $last_login = get_user_meta($user_id, 'last_login', true); //picking up wordpress date time format $date_format = get_option('date_format') . ' ' . get_option('time_format'); //converting the login time to wordpress format $the_last_login = mysql2date($date_format, $last_login, false); //finally return the value return $the_last_login; } //get current user object $current_user = wp_get_current_user(); //get the last login time by passing the user id to the above function. echo get_last_login($current_user->ID); ?>We are done. 🙂
works great, thanks